TUM dataset¶
This tutorial reads and visualizes an RGBDImage
from the TUM dataset [Strum2012].
5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 | # examples/Python/Basic/rgbd_tum.py
import open3d as o3d
import matplotlib.pyplot as plt
if __name__ == "__main__":
print("Read TUM dataset")
color_raw = o3d.io.read_image(
"../../TestData/RGBD/other_formats/TUM_color.png")
depth_raw = o3d.io.read_image(
"../../TestData/RGBD/other_formats/TUM_depth.png")
rgbd_image = o3d.geometry.RGBDImage.create_from_tum_format(
color_raw, depth_raw)
print(rgbd_image)
plt.subplot(1, 2, 1)
plt.title('TUM grayscale image')
plt.imshow(rgbd_image.color)
plt.subplot(1, 2, 2)
plt.title('TUM depth image')
plt.imshow(rgbd_image.depth)
plt.show()
pcd = o3d.geometry.PointCloud.create_from_rgbd_image(
rgbd_image,
o3d.camera.PinholeCameraIntrinsic(
o3d.camera.PinholeCameraIntrinsicParameters.PrimeSenseDefault))
# Flip it, otherwise the pointcloud will be upside down
pcd.transform([[1, 0, 0, 0], [0, -1, 0, 0], [0, 0, -1, 0], [0, 0, 0, 1]])
o3d.visualization.draw_geometries([pcd])
|
This tutorial is almost the same as the tutorial processing Redwood dataset. The only difference is that we use conversion function create_rgbd_image_from_tum_format
to parse depth images in the TUM dataset.
Similarly, the RGBDImage
can be rendered as numpy arrays:
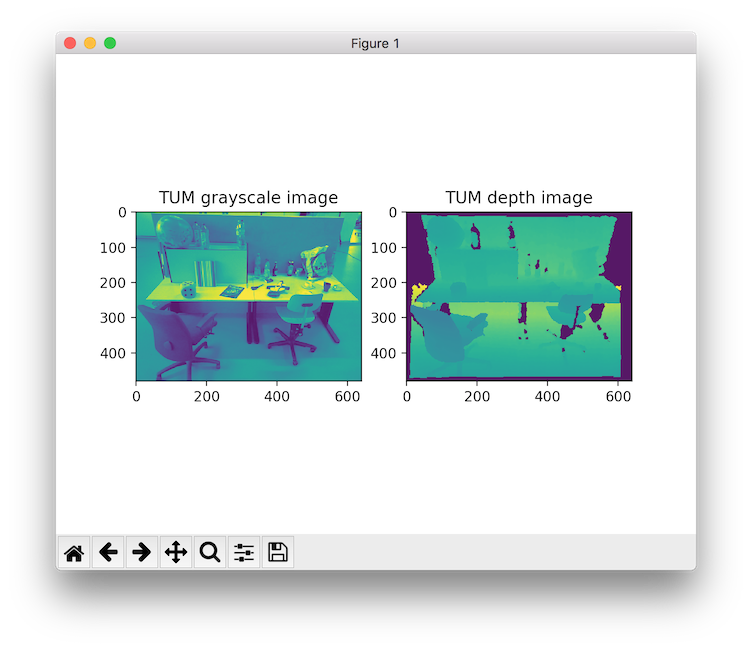
Or a point cloud:
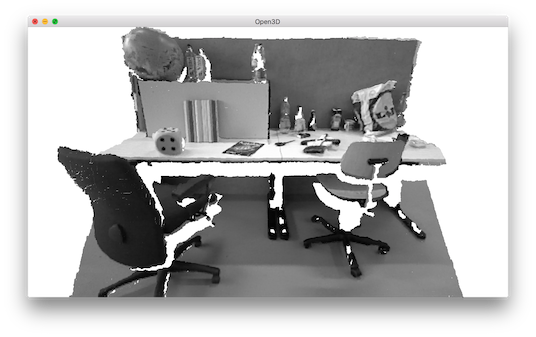